Javascript Object Notation (JSON) is a standard text-based format to structure data based on Javascript object syntax. We can see this format being used to transfer data in web applications.
{ "firstName": "Robert", "lastName": "Patrick", "age": 37, "address": { "streetAddress": "21, Glory Street", "city": "Boston", "state": "MT", "postalCode": "100-8888" }, "phoneNumbers": [ { "type": "home", "number": "212 555-4444" }, { "type": "office", "number": "212 555-2222" } ], "children": [], "spouse": null }
That’s many possibilities of using this format. This is very flexible and useful. Note that in one block of information we have all the relevant information about a person.
Loading a JSON file into an CSharp application
Consider a list containing information related to cars.
Model |
mpg |
cyl |
disp |
hp |
drat |
wt |
qsec |
vs |
am |
gear |
carb |
Normally we have this type of information listed on a flat file, but this time we will work with a JSON file format:
[ { "Model": "Mazda RX4", "mpg": "21", "cyl": "6", "disp": "160", "hp": "110", "drat": "3.9", "wt": "2.62", "qsec": "16.46", "vs": "0", "am": "1", "gear": "4", "carb": "4" }, { "Model": "Mazda RX4 Wag", "mpg": "21", "cyl": "6", "disp": "160", "hp": "110", "drat": "3.9", "wt": "2.875", "qsec": "17.02", "vs": "0", "am": "1", "gear": "4", "carb": "4" } ]
This is a simple example, with no sub-trees.
We will load this file into a CSHARP application, the first step is to create a csharp .NET wep application.
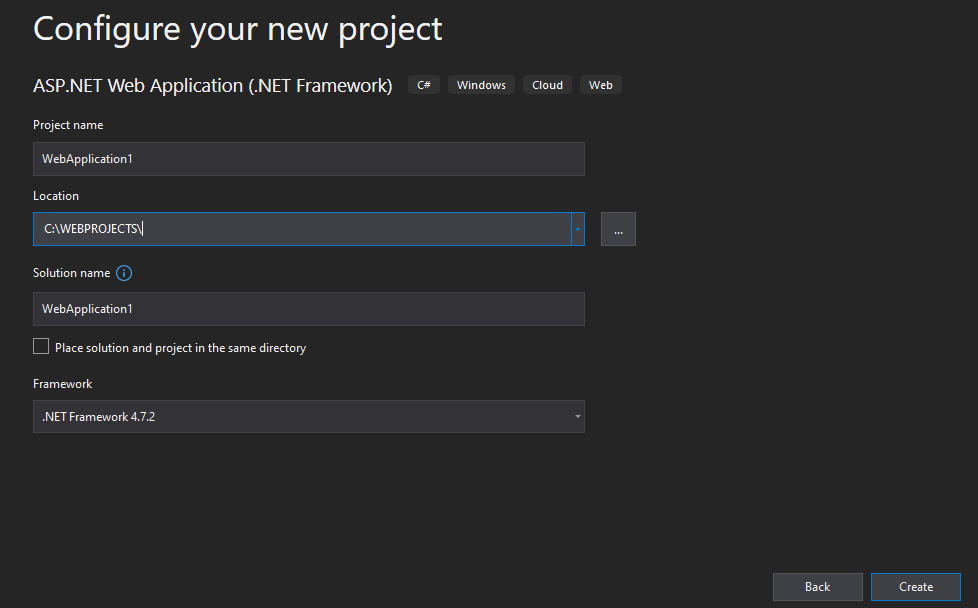
We could create a console application, a windows forms application or a Web Application. This time we will work with a Web Application.
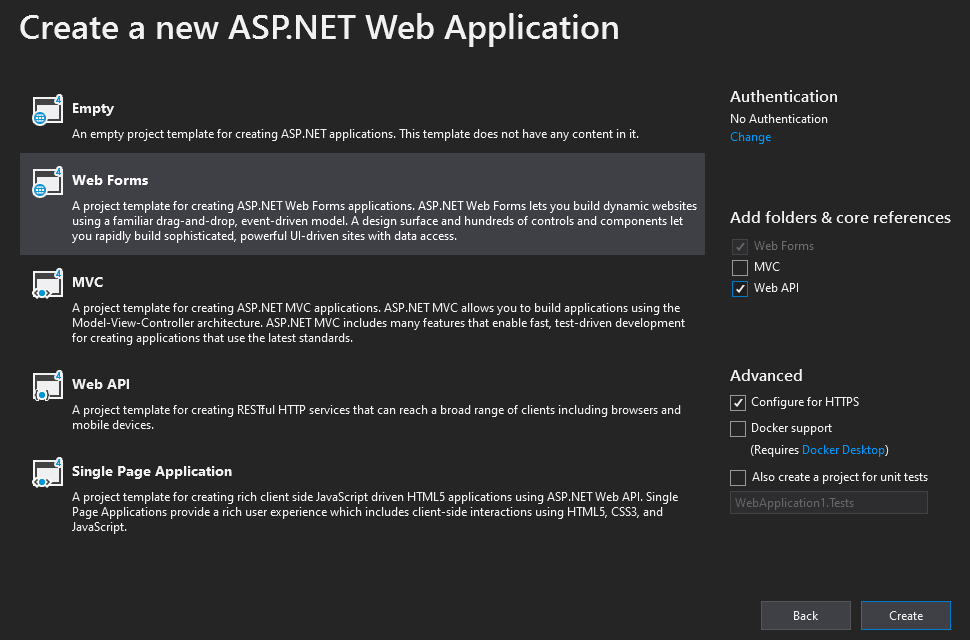
Now we have a Web Application project, but to work with JSON files, we’ll need a library to manipulate the JSON format.
Click on References and “Manage NuGet Packages”. Search for JSON, then the Newtonsoft.Json will appear. Select it and click on Install.

After that, add an ASPX page to the project.

On the csharp code, you should add the reference to the Newtonsoft.JSON library and add the code to open and use the JSON file.

See the code below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.IO;
using System.Data;
using WebApplication1.View;
using Newtonsoft.Json;
namespace WebApplication1.View
{
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
using (StreamReader r = new StreamReader("C:\\scripts\\Cars.json"))
{
string json = r.ReadToEnd();
var data = JsonConvert.DeserializeObject<List<Item>>(json);
How to store data on the application?
We need to add some data structure to store the data read from the JSON file.
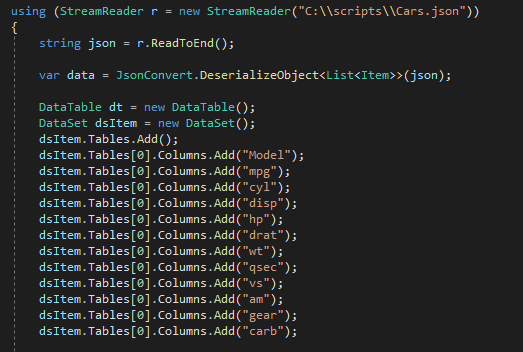
We can use a dataset. You can add the same columns of the JSON file.
How to show data on the application?
We need to add a visual object to show data. This time we will use a gridview.
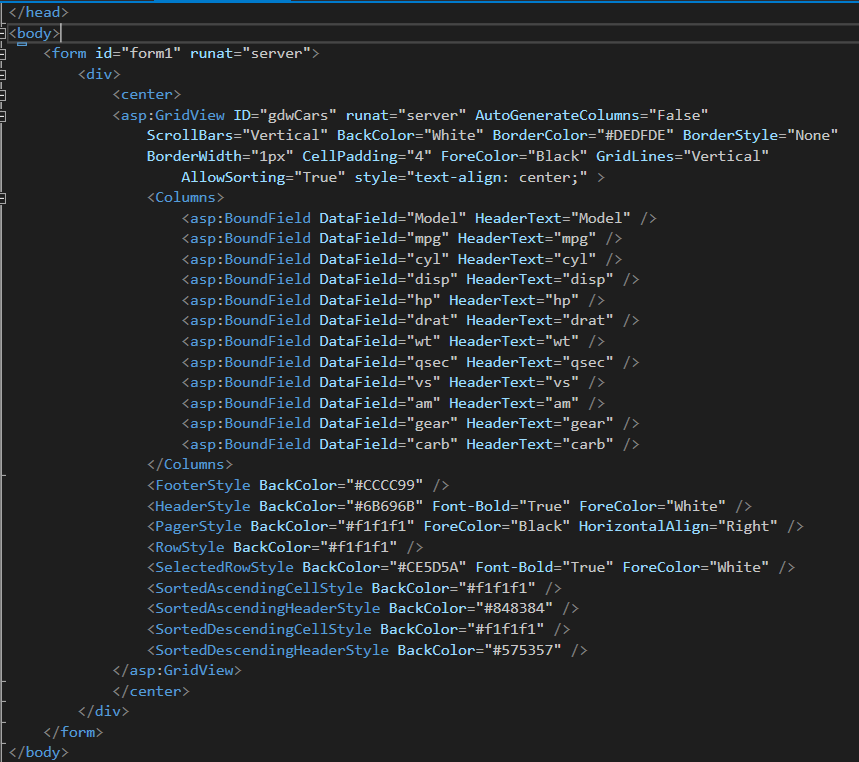
See the code below
Aspx code
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.View.WebForm1" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <center> <asp:GridView ID="gdwCars" runat="server" AutoGenerateColumns="False" ScrollBars="Vertical" BackColor="White" BorderColor="#DEDFDE" BorderStyle="None" BorderWidth="1px" CellPadding="4" ForeColor="Black" GridLines="Vertical" AllowSorting="True" style="text-align: center;" > <Columns> <asp:BoundField DataField="Model" HeaderText="Model" /> <asp:BoundField DataField="mpg" HeaderText="mpg" /> <asp:BoundField DataField="cyl" HeaderText="cyl" /> <asp:BoundField DataField="disp" HeaderText="disp" /> <asp:BoundField DataField="hp" HeaderText="hp" /> <asp:BoundField DataField="drat" HeaderText="drat" /> <asp:BoundField DataField="wt" HeaderText="wt" /> <asp:BoundField DataField="qsec" HeaderText="qsec" /> <asp:BoundField DataField="vs" HeaderText="vs" /> <asp:BoundField DataField="am" HeaderText="am" /> <asp:BoundField DataField="gear" HeaderText="gear" /> <asp:BoundField DataField="carb" HeaderText="carb" /> </Columns> <FooterStyle BackColor="#CCCC99" /> <HeaderStyle BackColor="#6B696B" Font-Bold="True" ForeColor="White" /> <PagerStyle BackColor="#f1f1f1" ForeColor="Black" HorizontalAlign="Right" /> <RowStyle BackColor="#f1f1f1" /> <SelectedRowStyle BackColor="#CE5D5A" Font-Bold="True" ForeColor="White" /> <SortedAscendingCellStyle BackColor="#f1f1f1" /> <SortedAscendingHeaderStyle BackColor="#848384" /> <SortedDescendingCellStyle BackColor="#f1f1f1" /> <SortedDescendingHeaderStyle BackColor="#575357" /> </asp:GridView> </center> </div> </form> </body> </html>
CSharp code
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.IO; using System.Data; using WebApplication1.View; using Newtonsoft.Json; namespace WebApplication1.View { public partial class WebForm1 : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { using (StreamReader r = new StreamReader("C:\\scripts\\Cars.json")) { string json = r.ReadToEnd(); var data = JsonConvert.DeserializeObject<List<Item>>(json); DataTable dt = new DataTable(); DataSet dsItem = new DataSet(); dsItem.Tables.Add(); dsItem.Tables[0].Columns.Add("Model"); dsItem.Tables[0].Columns.Add("mpg"); dsItem.Tables[0].Columns.Add("cyl"); dsItem.Tables[0].Columns.Add("disp"); dsItem.Tables[0].Columns.Add("hp"); dsItem.Tables[0].Columns.Add("drat"); dsItem.Tables[0].Columns.Add("wt"); dsItem.Tables[0].Columns.Add("qsec"); dsItem.Tables[0].Columns.Add("vs"); dsItem.Tables[0].Columns.Add("am"); dsItem.Tables[0].Columns.Add("gear"); dsItem.Tables[0].Columns.Add("carb"); int cont = 0; foreach (WebApplication1.View.Item item in data) { DataRow dr = dsItem.Tables[0].NewRow(); dr["Model"] = item.Model.ToString(); dr["mpg"] = item.mpg.ToString(); dr["cyl"] = item.cyl.ToString(); dr["disp"] = item.disp.ToString(); dr["hp"] = item.hp.ToString(); dr["drat"] = item.drat.ToString(); dr["wt"] = item.wt.ToString(); dr["qsec"] = item.qsec.ToString(); dr["vs"] = item.vs.ToString(); dr["am"] = item.am.ToString(); dr["gear"] = item.gear.ToString(); dr["carb"] = item.carb.ToString(); dsItem.Tables[0].Rows.Add(dr); } //GridView1; gdwCars.DataSource = dsItem; gdwCars.DataBind(); int i = 0; } } } }
Compile and build the entire solution to execute the application to see the results.
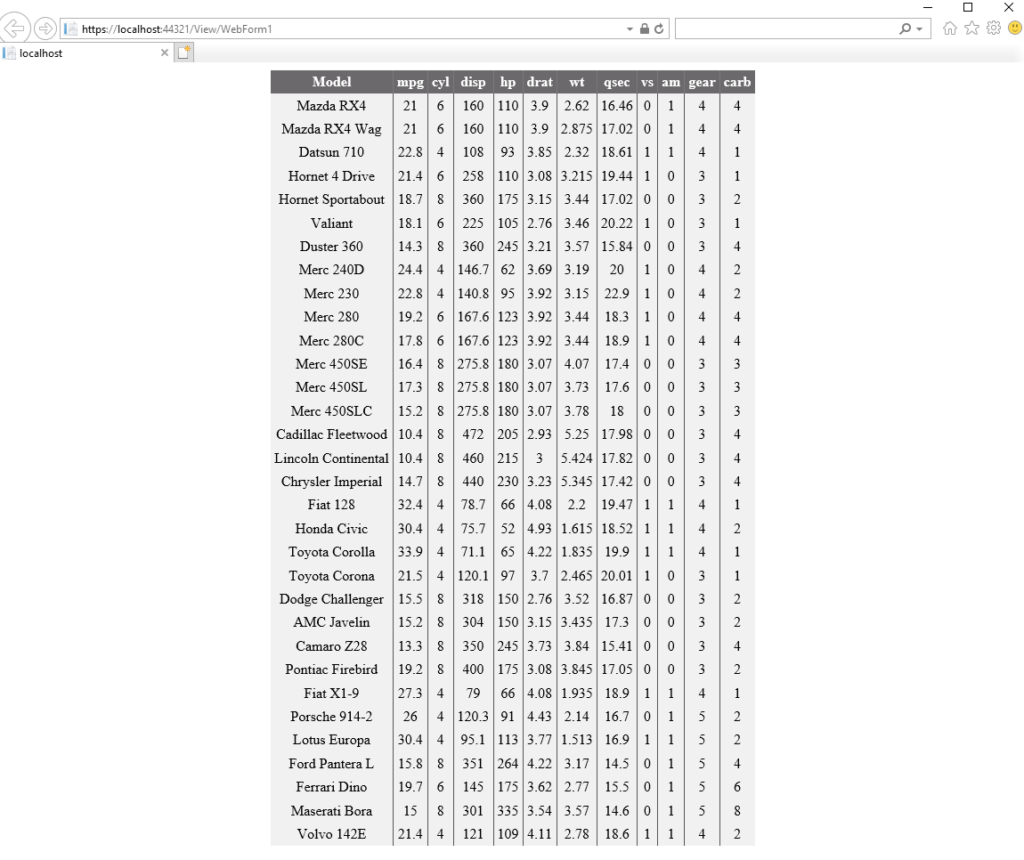
That’s the result list. This way we can see the data extracted from JSON file in a aspnet page.
I hope this is useful to you. Have a good day.